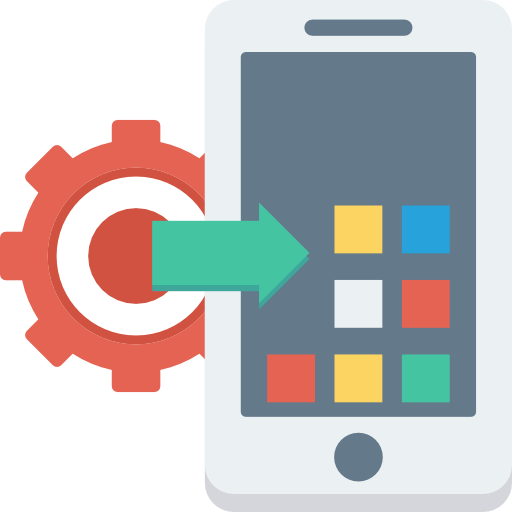
Arguments and Interface in Javascript
Let’s start with a simple function person
:
function person(name, sex, age){ |
If we want to add new parameters height
and weight
, we need to declare them one by one first:
// declare "height" and "weight" |
Adding more parameters leads to less comprehensibility. To make things worse, when working on fundamental toolkits, it’s possible that the sequential order of parameters can not be guaranteed.
Then, how to create a function with uncertain amount/order of parameters?
Arguments
Some say that JavaScript is a tricky language, and arguments
is a tricky part of it.
function student(){ |
Output:
arguments : ["kevin", "john", "lucy"] |
It seems that arguments
is an array(but actually it is not, explains afterwards), containing all parameters imported. With arguments
introduced, we could make functions with uncertain parameters as following example.
function student(){ |
Output:
4 : david |
But when we use pop
or push
method to arguments
:
function student(){ |
Output:TypeError: Object #<Object> has no method 'push'
It will throw an error – that means arguments
is not an array but an Object quite similar. We should use a little trick here, to get a native array:
function student(){ |
Output:["kevin", "john", "lucy", "mike", "david", "lili"]
A more complicated version is a template format
function:
function format() { |
Output:"daddy loves katty, mummy loves katty too."
The previous mode which puts every parameters in an array, we could call it SEQUENCE
. Is it good enough?
// 2006-03-01: "name", "gender", "age" |
It really happens: with the booming of project, length of arguments increasing, the engineers are hard to distinguish parameters from each other. We could not know what they stand for unless referring to comments or looking into person
function. Less self-explainable parameters make it hard to maintain.
JSON
A better solution JSON
:
// Migrate to JSON |
First benefit is that parameters become much more self-explainable, therefore developers do not need to dig into the function or even set breakpoints to find out their meanings then back to work.
What’s more, when adding a new parameter, there is no need to worry about the order.
Interface
Unlike other OO program languages like Java
, javascript does not have any built-in interface
. Although the implementation of interface in javascript is still an arguable point, it is a very flexible language and could be applied with interface without much efforts.
Like Interface
ensures the function has all required methods
, the following class is used to ensure the called function contain all the necessary parameters, if not then throw an error.
// Add 'validate' method to an object to be checked |
For example, we use validate
method to check person
:
jay = { |
It is definitely possible to enrich the validation functions:
// New a person object and apply validate method |
Moreover, if we change the return this.obj
with return this
and add a new getter function, the methods could be applied in a chain style:
var candidate = $(jay).validate({...}) |
I will recommend Pro JavaScript Design Patterns for its chapter on interface
concept and comparison of design patterns, between other languages and javascript.